Tech Demos
Some examples of technical demonstrations I've created. All made as solo course assessment projects, these were each used to learn a new concept in a custom environment, before applying the concept in a more advanced manner using the Unity engine.
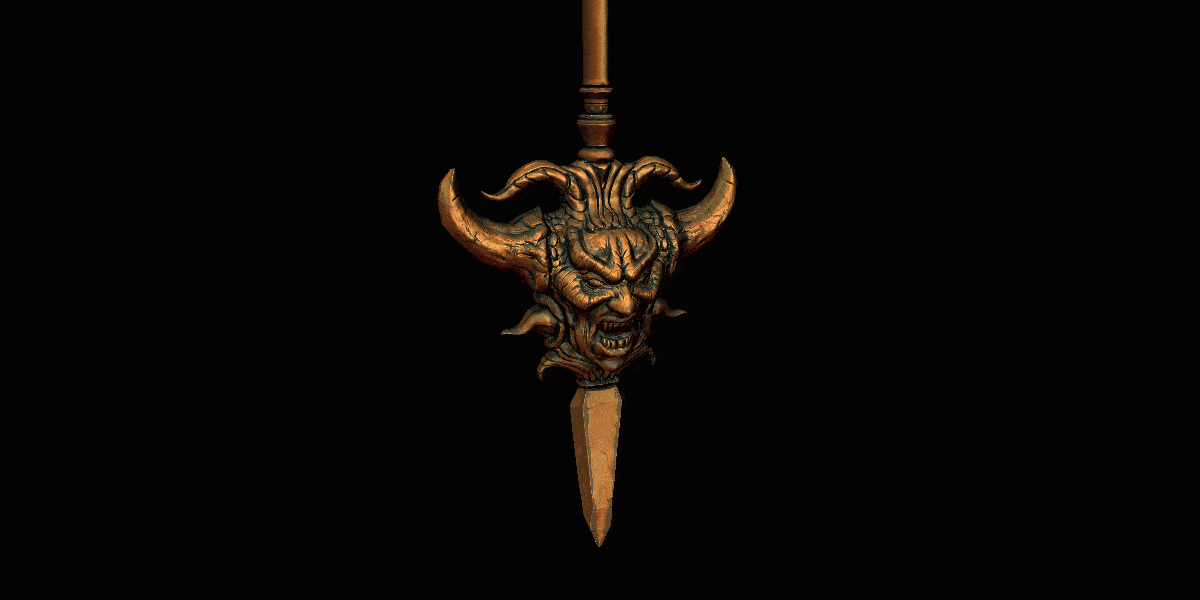
OpenGL Graphics Renderer
Description
This project was created as part of an assessment to create a basic custom graphics engine using OpenGL.
It uses a projection matrix camera, with first-person flying controls, which can be used to inspect a spinning mesh with coloured light sources,
detailed with albedo, normal and specular maps.
While my implementation isn't a comprehensive game engine by any means, it gave me a fundamental understanding about aspects of the graphics pipeline
and custom vertex/fragment shaders,
as well as technical aspects of how 3D assets work, and asset management.
Developing this lead into further experimentation with advanced shaders with Unity's Shader Graph, as seen below.
Implementation
Written in C++ using the GLFW and GLM OpenGL libraries. 3D mesh files and their 2D textures are loaded into
RAM/VRAM at runtime and stored as a mesh/object class, which can have its transform matrix properties manipulated.
The basic Lit shader is written using GLSL.
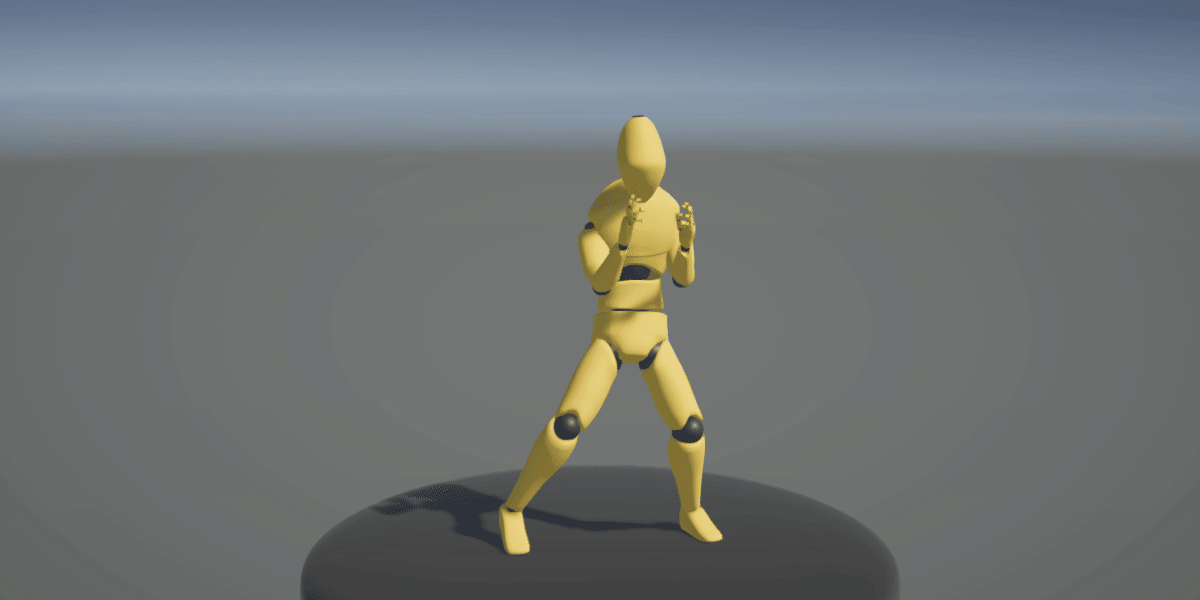
Unity Shaders and Animation Project
Description
This project was an experimentation with ShaderGraph functionality built into the Unity engine, as well as Animation blend trees,
and how the two integrate with a C# Unity codebase.
It contains a set of 6 shaders I created to alter the appearance of a 3D animated character, and the ability to select 6 different
animations with callbacks - such as a sit-ups animation with a UI counter.
This project gave me familiarity with more technical aspects of the Unity engine that I hadn't interacted with prior.
Implementation
Except for basic Lit/Unlit, each shader was created using Shader Graph. Animation assets were imported and played back using
a custom animation controller and blend tree, including additional un-selectable random idle events, and transitional animations.
Unity's Animation Events system was used to drive various callbacks.
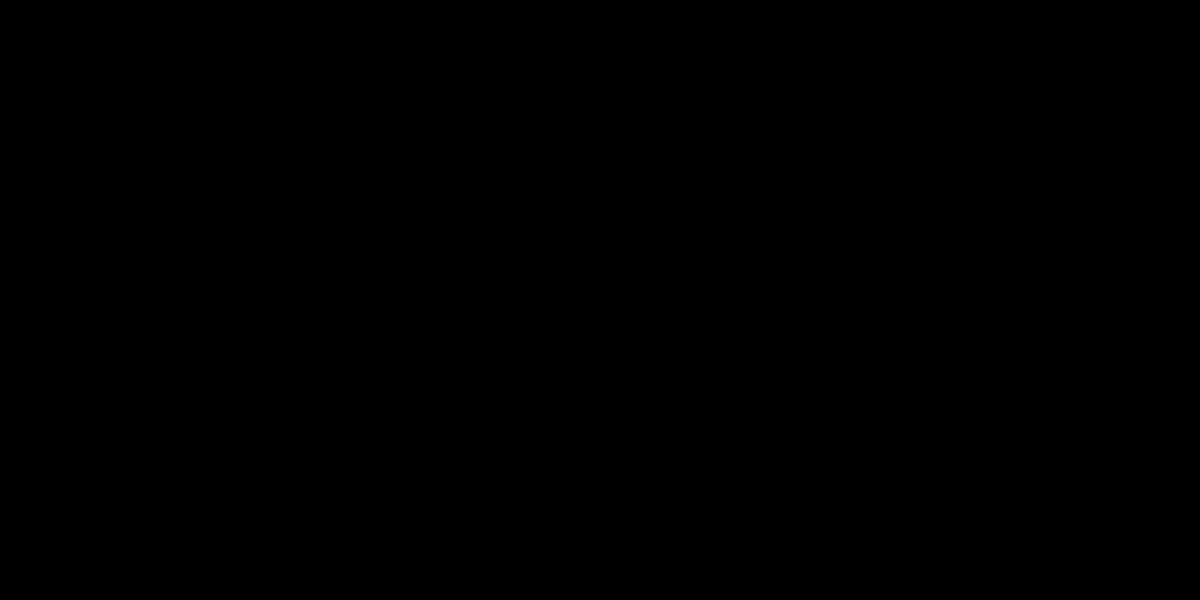
2D Rigid Body Physics Demonstration
Description
This project was created as an exercise in physics and collision detection with different shapes.
You are able to place static and dynamic squares, circles or planes and marvel at the the way in which shapes avoid being inside one another.
The experience of physics simulations in a 2D environment utilized in this project was the inspiration for me to experiment further with customized 3D physics within Unity in the following project.
Implementation
Custom physics and collision simulation was written using C++, again using OpenGL rendering libraries, and ImGui for text.
I implemented collision detection and resolution for each potential pair between static and dynamic squares, circles and planes.
A build and some of the code for this project is available on the Code Examples page!
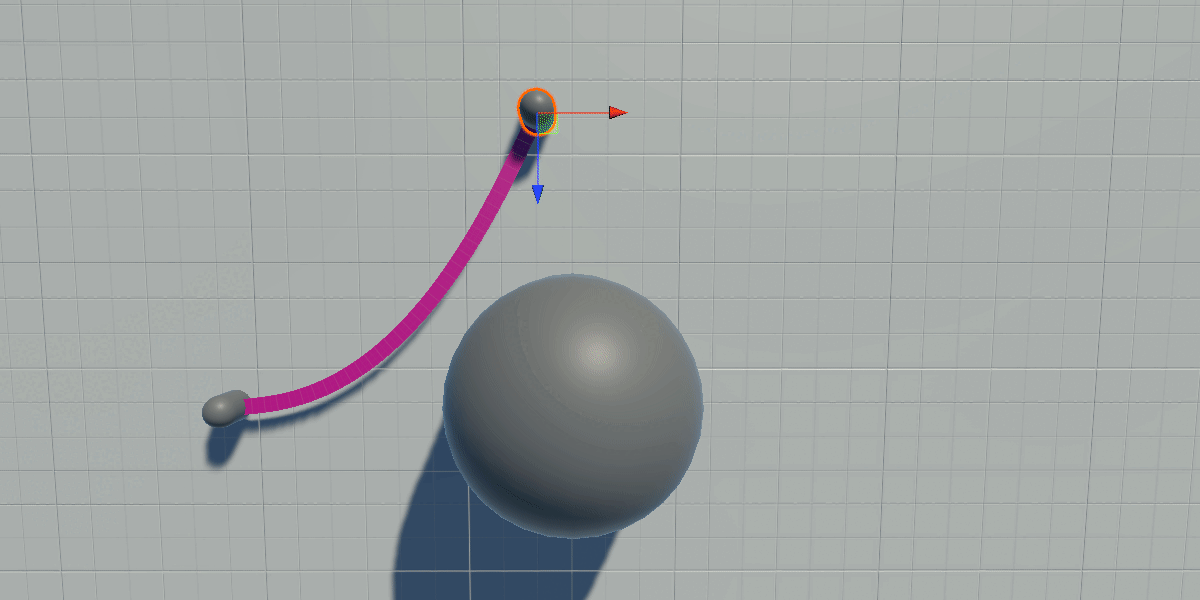
Rope Simulation with Verlet Integration
Description
My goal for this project was to create a physically accurate rope/cable, and was inspired by rope physics in recent Naughty Dog games.
In addition to the rope system itself, I created a basic playground environment to be able to attach and detach ends of a rope to static or dynamic rigid bodies,
in order to demonstrate how physical bodies affect the rope, and how its tension affects them back. This was sufficient, but with the surprising ease in which
verlet integration solved basic rope simulation, I experimented with adding additional features, which have enabled me to use the system in other projects,
particularly visual flair.
Taking it further
Integrating breakable ropes felt like the logical next step to me, as the rope was already being simulated via nodes 'strung' together.
I integrated a system to manually cut ropes, or cause them to break (in roughly the middle, or the spot most under tension) when
the differentation between nodes exceeds a threshold.
In addition, as I wanted to use this asset for visual flair in other projects, I integrated a smoothing algorithm to be able to simulate ropes using very
few nodes, whilst keeping the appearance high fidelity. I also implemented real-time mesh generation for cases where using using a textured line with billboarding
is insufficient.
I'm still working on this project, and intend to add more features to make it viable to be used as more than visual flair in future games.
Implementation
Using a custom C# script, my rope simulation was built in the Unity engine. Several parameters allow tweakable appearance (radius, smoothing, etc.), simulation (tension, length, tearing, etc.), and
external forces (optionally locking ends to a transform, attaching a transform or rigid body anywhere on the rope, etc.).
The rope is simulated using a list of 'nodes' that store only their previous and current position, from which everything else is derived. As well as being affected (or not) by gravity,
nodes push and pull on one another by caculating their delta from a pre-calculated segment length, while also depenetrating themselves from any colliders.
For rendering, the rope can be smoothened using Chaikin's corner-cutting algorithm to generate additional 'nodes' for rendering only. The node positions can be output to Unity's Line Renderer component,
or optionally used to generate a cylindrically-shaped mesh on a fixed update loop.
The code for this project is available on the Code Examples page!